The following exercises are taken from chapter 8 of the textbook.
Exercise 8.4 Open the project dome-v2. This project contains a version of the DoME application rewritten to use inheritance, as described above. Note that the class diagram displays the inheritance relationship. Open the source code of the DVD class and remove the ‘extends Item‘ phrase. Close the editor. What changes do you observe in the class diagram? Add the ‘extends Item‘ phrase again.
Exercise 8.5 Create a CD object. Call some of its methods. Can you call the inherited methods (for example, setComment)? What do you observe about the inherited methods?
Exercise 8.6 In order to illustrate that a subclass can access non-private elements of the superclass without any special syntax, try the following slightly artificial modification to the CD and Item classes. Create a method called printShortDetails in the CD class. Its task is to print just the artist and the title of a CD on a line by itself. However, because the titIe field is private in the Item class, it will be necessary to add a public getTitIe method to Item. Call this method from printShortDetails to access the title for printing. Remember that no special syntax is required when a subclass calls a superclass method. Try out your solution by creating a CD object. Implement a similar method in the DVD class to print just the director and title.
Exercise 8.8 Open the dome-v2 project. Add a class for games and a subclass for video games to the project. Create some video game objects and test that all methods work as expected.
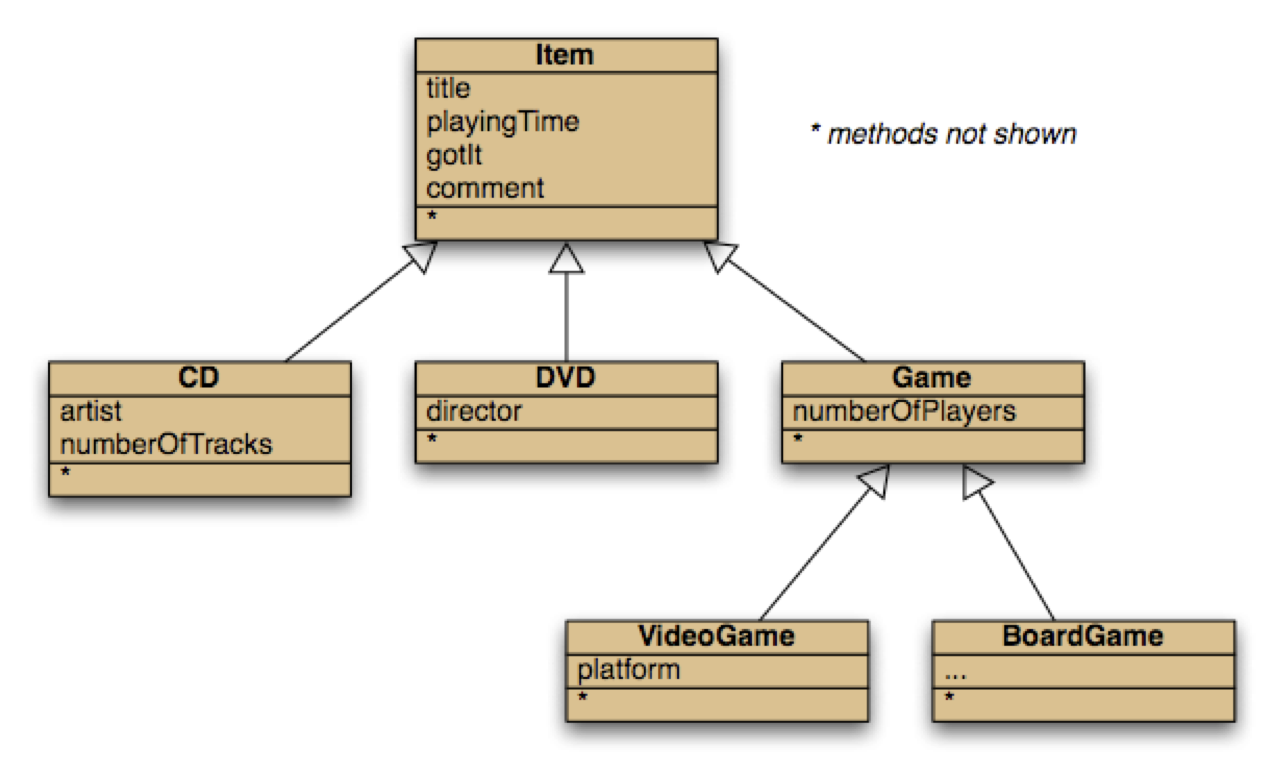
Exercise 8.12 Assume we have four classes: Person, Teacher, Student and PhDStudent. Teacher and Student are both subclasses of Person. PhDStudent is a subclass of Student.
a. Which of the following assignments are legal, and why or why not?
Person p1 = new Student();
Person p2 = new PhDStudent();
PhDStudent phd1 = new Student();
Teacher t1 = new Person();
Student s1 = new PhDStudent();
b. Suppose that we have the following legal declarations and assignments
Person p1 = new Person();
Person p2= new PerSon ();
PhDStudent phd1 = new PhDStudent();
Teacher t1 = new Teacher () ;
Student s1 = new Student();
Based on those just mentioned, which of the following assignments are legal and or why not?
s1 = p1;
s1 = p2;
p1 = s1;
t1 = st;
s1 = phd1;
phd1 = s1;
Exercise 8.13 Test your answers to the previous question by creating bare-bones versions of the classes mentioned in that exercise and trying it out in BlueJ